Browser Router Vs Hash Router in React JS
- Pradeep Kumar
- Jun 1, 2020
- 3 min read
Updated: Sep 28, 2020
This blog is all about explains about the following things
What is routing in React Js and why it is needed for React Js application?
Why Hash Router is used for real time projects rather than a Browser Router?
What is routing in React Js and why it is needed?
React Js is used to develop a single page application where it contains a single index.html file and each component of React js renders inside the index.html file on div element
React js uses a virtual DOM so that if a browser is refreshed the DOM will be reloaded, hence the main parent component will be rendered on reloading the page
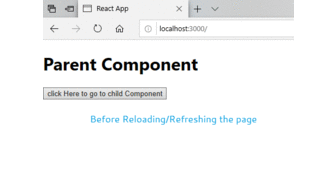
In the above GIF image on reloading/refreshing it redirects to the parent component
To avoid these developers use React js Routing which is an npm package. So, that users can see a proper definition of the component at the URL and users can directly load the child component on reloading the page.
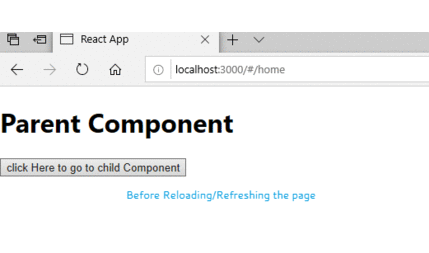
In the above GIF image on reloading/redirecting it stays at the child component
So many of the developers use routing either "Browser Router" or "Hash Router".
Browser Router vs Hash Router
If a website is build with Browser Router for React Js application the website URL is probably be like : "https://www.techblogsnews.com/"
if a website is build with Hash Router for React Js application the website URL is probably be like: "https://www.techblogsnews.com/#/"
You will see an "#" is appended at the end of the URL for Hash Router.
if you are switching from component to component in React js application in production environment after deploying it in a production server .
For Browser Router let us say "https://www.techblogsnews.com/" to "https://www.techblogsnews.com/Blog" then the server may throw an ERROR "404: Page Not Found." these is because the server might be searching for folder Blog which contains an HTML file.
To avoid these to indicate that our entire application is rendered in a single page index.html Hash Router is used which appends the "#" at the end of the main domain will indicate the sever that the HTML code will be loaded at index.html file
So, on these note Hash Router are prefer-ed than Browser Router at production level
Sample React Js Application using Hash Router
Use the npx create-react-app <appname> command to create sample react application.
After creating the template override the App.js file as per your requirement
install react-router-dom npm package of v5
Here I am using history npm package of v4 to use browser location
App.js
import React from 'react';
import './App.css';
import {HashRouter as Router,Route,Switch,Link,useHistory,Redirect} from 'react-router-dom';
import Home from './Home';
import {createHashHistory} from 'history';
import Test from "./Test"
export const history = createHashHistory()
class App extends React.Component{
constructor(props){
super(props);
}
componentDidMount(){
}
render(){
return(
<div>
<Router>
<Route exact path="/home" ><Home/></Route>
<Route exact path="/home/test"><Test/></Route>
</Router>
</div>
);
}
}
export default App;
Now create child components in my case it is Home & Test
Home.js
import React from 'react';
import './App.css';
import {HashRouter as Router,Route,Switch} from 'react-router-dom'
import {history} from './App'
import Test from "./Test"
class Home extends React.Component{
constructor(props){
super(props);
this.handleClick = this.handleClick.bind(this);
}
handleClick(){
history.push("/home/test");
}
componentDidMount(){
}
render(){
return (
<div>
<h1>
Parent Component
</h1>
<button onClick={this.handleClick}>click Here to go to child Component</button>
</div>
)
}
}
}
export default Home;
Test.js
import React from "react"
class Test extends React.Component{
constructor(props){
super(props);
}
render()
{
return <div>testComponent </div>
}
}
export default Test;
Note
In my application Test component is child component of Home component and Home component is child component of App component. So, Home component is parent component of Test component.
Thanks & Regards,
PRADEEP KUMAR BAISETTI
Associate Trainee – Enterprise Application Development
Digital Transformation
Thank you very much for sharing, but your analysis is wrong
Deep explaination.
Awesome blog
http://www.traininginchennai.co.in/sap-training-in-chennai/
It's interesting I think I have to go through it again since there was lot of things explained that I didn't know because I don't really know much about tech.
Nice